Introduction to Laravel Development Features Benefits
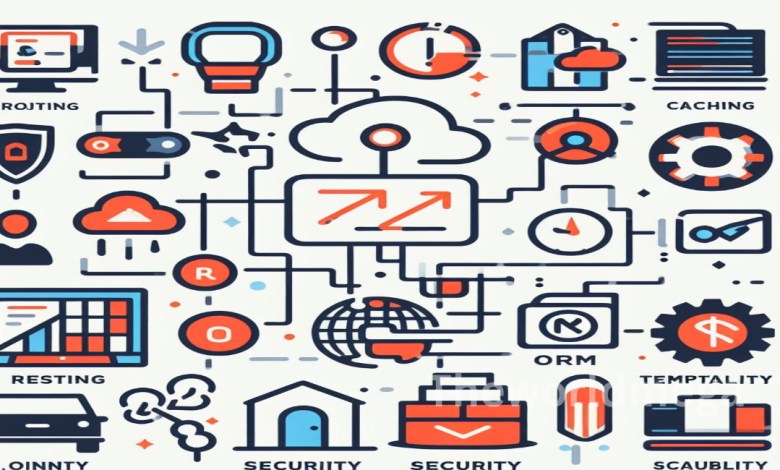
With its inception, Laravel an open-source PHP web application framework has become popular. With its elegant code syntax, robust built-in tools, and abundant ecosystem, Laravel has become a go-to choice for developing a wide variety of web and mobile applications. This blog will share an insight on the important capabilities, and benefits of using Laravel as your framework for web development projects.
What is Laravel?
Laravel is a free, open-source PHP web framework created by Taylor Otwell in 2011. It follows the model-view-controller (MVC) architectural pattern and is intended to make common web development tasks like authentication, routing, sessions, and caching simpler and faster using PHP.
Some significant features include
Expressive Routing
Defines clean URL structures and routes requests to appropriate handlers. Supports RESTful architecture out-of-the-box.
Blade Templating Engine
Renders frontend templates using simplified PHP syntax to keep presentational code separate from business logic.
Eloquent ORM
Provides a beautiful Active Record implementation for working with database tables as model objects.
Middleware
Layers for filtering and processing requests before they reach route handlers. Useful for authentication, logging, compression, and more.
Service Container
Central container for managing class dependencies through inversion of control and promoting loose coupling between components.
Composer Support
Deep integration with Composer dependency manager makes including third-party libraries a breeze.
Artisan CLI
Powerful command line interface for managing migrations, queues, caching, tests, and other application tasks.
Event System
Dispatching of job events allows decoupling different components across the app.
Testing Suite
Integrated unit and feature testing framework for performing tests at code and browser levels.
Laravel aims to find a balance between developer experience and architectural flexibility. It speeds up many tedious coding tasks while giving freedom in how the application pieces fit together.

Advantages of Laravel
There are many key advantages to using the Laravel framework for web application development. To harness the benefits offered by Laravel you can hire Laravel developers with expertise in PHP development.
Expressive, Intuitive Syntax
Laravel uses easy to read code inspired by frameworks like Ruby on Rails. Very natural compared to some other PHP options.
Batteries Included Architecture
Many essential tools like the Eloquent ORM, routing, caching, and authentication are built-in, allowing faster development.
Comprehensive Documentation
Extremely detailed documentation with many code examples helps quickly get developers up to speed.
Active Community
As one of the most popular PHP frameworks, Laravel has a wealth of tutorials, plugins, and learning resources created by its community.
Highly Scalable
Modular design using contracts makes Laravel very extendable for small personal projects up through enterprise systems.
Time Saving Features
Built-in authentication, mailing, testing, queues and more allow concentrating effort on app logic and UX.
Third Party Integrations
Easy integration with tools like Redis, Memcached, Elasticsearch, AWS S3, Slack etc. via first party and community packages.
High Performance
Caching, queue workers, optimized code traces result in applications that are very fast and responsive out-of-the-box.
Security Conscious
Protection against common vulnerabilities like SQL injection, XSS, and CSRF attacks built right into the core framework.
For lean development teams looking to build stable and secure PHP web applications rapidly, Laravel’s capabilities can significantly accelerate the process.
When to Use Laravel
Laravel is well-suited for a variety of web development use cases:
Custom CRM, CMS, or LMS Platforms
Laravel is ideal for SaaS applications thanks to its modular architecture. Integrations are easy to add.
Web Applications with Heavy Database Usage
The Eloquent ORM makes it simple to be productive when building complex database models and relations.
Applications Requiring API Development
Building robust APIs is seamless in Laravel with routing, authentication, rate limiting, and testing features.
Web Apps with Real-Time Functionality
Laravel Echo makes integrating web sockets with channels easy for real-time event-driven apps.
Teams with Previous PHP Experience
Laravel is easy to learn for PHP developers and reduces time writing boilerplate code.
Startup MVPs Needing Rapid Iteration
Laravel’s scope lends itself well to quickly building and launching early product versions.
Sites Handling Lots of Traffic
Built-in caching, queues, and rate limiting allows Laravel projects to scale efficiently.
Secure Applications
Laravel’s threat protection mitigates risks right out of the box.
While suitable for many use cases, Laravel may not be the right choice when minimalism or simplicity is paramount. But it offers a full-featured framework for most web application projects.
Also Read: What Changed? Axis Bank Devalues 5 Credit Cards
Installing Laravel
The Laravel framework has a few system requirements:
- PHP >= 7.3
- BCMath PHP Extension
- Ctype PHP Extension
- Fileinfo PHP Extension
- JSON PHP Extension
- Mbstring PHP Extension
- OpenSSL PHP Extension
- PDO PHP Extension
- Tokenizer PHP Extension
- XML PHP Extension
It’s also recommended to have Composer, which is a dependency manager for PHP. Composer can be downloaded from getcomposer.org and installed globally on the system.
Once PHP and Composer are set up, Laravel can be installed in a few easy simple steps:
1. Open the command line terminal and create a new Laravel project using Composer:
composer create-project laravel/laravel project-name
2. This will kick off the Composer installer to create a fresh Laravel installation in the specified directory.
3. Once complete, switch to the application directory:
cd project-name
4. Run the local development server using the Artisan command line:
php artisan serve
This will start the built-in PHP web server at localhost:8000 by default.
5. Open a browser and navigate to http://localhost:8000 to see the Laravel welcome page.
That’s all that is required to get a new Laravel project up and running! The full framework with routing, migrations, build tools and more is ready build your application.
Laravel Application Structure
The default Laravel project structure follows common MVC patterns:
- app – Core application code like controllers, models, middleware
- bootstrap – Framework bootstrapping and configuration
- config – Application configuration settings
- database – Database migration and seeds
- public – Front-facing assets like images, CSS, JavaScript
- resources – Views, translation files, assets
- routes – Application route definitions
- storage – Caches, compiled assets, logs, sessions
- tests – Automated application tests
- vendor – Composer dependencies
The app directory contains subfolders like Http, Console, Providers, Models and more based on typical MVC partitioning. When first diving into a new Laravel project, it’s easiest to focus on the routes definitions, controllers, and resource views to build out initial application pages and logic.
Also Read: Anonymous Crypto Wallet 10 Compelling Reasons for Using
Routes & Controllers
Let’s look closer at routes and controllers which handle mapping requests and providing responses.
Routes are defined in routes/web.php. This file maps URL patterns to closures or controllers:
Route::get(‘/about’, function() {
return view(‘about’);
});
Route::get(‘/contact’, ‘ContactController@show’);
For simple routes, a closure can render a view directly. For more complex logic, a controller is preferred.
Controllers group related request handling code into a single class:
class ContactController extends Controller {
public function show() {
return view(‘contact’);
The controller can depend on other services or repositories to build the response.
Conventionally, controllers are stored under the app/Http/Controllers directory. The Artisan CLI tool can quickly generate them:
php artisan make:controller ContactController
This creates the controller stub ready for adding methods like show(). Together, routes and controllers are the cornerstone of Laravel request flow.
Blade Templating
When controller logic needs to render actual HTML content, Laravel uses the Blade templating engine.
Blade view files have a.blade.php extension and reside in the resources/views directory.
Here is an example contact.blade.php view:
@extends(‘layouts.app’)
@section(‘content’)
<h1>Contact Us</h1>
<p>Call us at 555-555-1234</p>
@endsection
Views can extend layouts which define common markup like headers and footers. Sections delimit content for the page.
Blade also provides shortcuts for control structures like loops and conditionals:
@if($user)
// user is logged in
@else
// user is guest
@endif
With this templating layer, presentation markup stays separate from application code. Views accept data to render.

Authentication System
User management and authentication are required for many web applications. Laravel provides simple ways to implement login, registration, password reset, email verification, and more out-of-the-box.
The make: auth Artisan command will scaffold all the routes, views, and controllers needed for basic authentication:
php artisan make: auth
With very little code, you can have a custom Tailwind CSS-driven interface allowing user signup, login, and authentication across application views and controllers.
Laravel authentication integrates directly with the Eloquent ORM making user management seamless. Defining authorization rules is simple as well using middleware and gates.
Conclusion
Laravel provides an intuitive, full-featured framework for PHP web development. Some key strengths are its expressive syntax, time-saving features like authentication and queues, and easy integration with databases and third-party tools.
For dedicated development team already comfortable with PHP, Laravel is easy to install and start building applications quickly. The learning curve is gentle thanks to comprehensive documentation and a vibrant community. With its modular design and robust ecosystem, Laravel excels at small personal projects up through complex enterprise systems. If you’re looking for a modern, elegant way to build PHP web apps, Laravel is worth your consideration and makes an excellent choice of framework for